Overview
Basic Input/Output
The provided mappings define how specific LUC keywords are transpiled into C# equivalents, along with their necessary parameters and dependencies. These mappings ensure that LUC commands integrate seamlessly with C#, making the language intuitive while maintaining robust functionality.
„print“: Maps to Console.WriteLine
, allowing output to the console. It requires a parameter for the message to display and includes using System
for access to the .NET framework. For Example:
print("Hello, World!");
„read“: Maps to Console.ReadLine, enabling input from the console. It also requires a parameter and includes using System.
userInput := read();
print("You entered: " + userInput);
„read_next_input“: Maps to Console.ReadKey, which waits for and retrieves the next key press from the user. This also depends on using System.
print("Press any key to continue...");
read_next_input();
String Methods
The mappings define how LUC keywords correspond to C# methods for string and object operations, making LUC intuitive and aligned with C#’s robust functionality. Here are some examples:
„len“: Maps to Count
, used to determine the number of elements in an object.
numbers := [1, 2, 3, 4, 5];
print(len(numbers));
„upper“: Maps to ToUpper, converting text to uppercase.
text := "hello";
print(upper(text));
„begins_with“: Maps to StartsWith
, verifying if a string starts with specific characters.
word := "Lucid";
print(begins_with(word, "Lu"));
„join“: Maps to string.Join, combining elements into a single string (requires using System).
words := ["Hello", "World"];
print(join(" ", words));
Math Methods
This list highlights how LUC keywords are mapped to C# Math methods, enabling precise mathematical computations while simplifying syntax. Here’s an overview:
„absolut_value“: Maps to Math.Abs
, calculating the absolute value of a number.
print(absolut_value(-10));
„max“: Maps to Math.Max
, finding the larger of two values.
print(max(10, 20));
„exponential“: Maps to Math.Exp
, calculating the exponential value (e^x) of a number.
print(exponential(2));
„atan“: Maps to Math.Atan
, calculating the arctangent of a value, returning the angle in radians.
print(atan(1));
List/Collection Methods
This list demonstrates how LUC keywords correspond to C# list operations via methods from System.Collections.Generic
. It simplifies working with lists while leveraging C#’s robust functionality. Here’s an overview:
„add_to_list“: Maps to Add
, appending an item to the list.
myList := [1, 2, 3];
add_to_list(myList, 4);
print(myList);
„remove_from_list“: Maps to Remove
, deleting the first occurrence of a specified item from the list.
remove_from_list(myList, 2);
print(myList);
„sort“: Maps to Sort
, sorting the list in ascending order.
myNumbers := [3, 1, 2];
sort(myNumbers);
print(myNumbers);
„find“: Maps to Find
, locating the first item that matches a specified condition.
print(find(myList, x -> x == 3));
LINQ Methods
This list highlights LUC keywords mapped to C# LINQ methods, enabling concise and expressive queries on collections. LINQ (Language Integrated Query) provides powerful data manipulation capabilities. Here’s an overview:
„select“: Maps to Select
, transforming elements in a collection.
squared := select(myList, x -> x * x);
print(squared);
„where“: Maps to Where
, filtering elements based on a condition.
filtered := where(myList, x -> x > 2);
print(filtered);
„count“: Maps to Count, counting elements in a collection.
print(count(myList));
„max_of_list“: Maps to Max
, retrieving the maximum value from a collection.
print(max_of_list(myNumbers));
File and Directory Methods
This list demonstrates LUC keywords mapped to C# file and directory handling methods, offering streamlined interaction with the file system via System.IO
. Here’s a quick guide:
„read_file“: Maps to File.ReadAllText
, reading the entire content of a file.
content := read_file("example.txt");
print(content);
„write_file“: Maps to File.WriteAllText
, writing content to a file (overwrites if exists).
write_file("example.txt", "Hello LUC!");
„create_directory“: Maps to Directory.CreateDirectory, creating a new directory.
create_directory("NewFolder");
„list_directories“: Maps to Directory.GetDirectories, retrieving a list of subdirectories within a directory.
dirs := list_directories(".");
print(dirs);
Dictionary Methods
This list of LUC keywords corresponds to C# dictionary operations using System.Collections.Generic
, providing intuitive commands for managing key-value pairs in dictionaries. Here’s a breakdown:
„add_to_dictionary“: Maps to Add
, which adds a new key-value pair to the dictionary
dict := {};
add_to_dictionary(dict, "name", "Paul");
print(dict);
„remove_from_dictionary“: Maps to Remove
, which removes a key-value pair by its key.
remove_from_dictionary(dict, "name");
print(dict);
„dictionary_contains_value“: Maps to ContainsValue
, checking if a specific value exists in the dictionary.
add_to_dictionary(dict, "age", 19);
print(dictionary_contains_value(dict, 19));
„get_dictionary_values“: Maps to Values
, retrieving a collection of all values in the dictionary.
values := get_dictionary_values(dict);
print(values);
Date and Time Methods
This set of LUC keywords corresponds to date and time operations in C#, leveraging the DateTime
structure and other related methods. Here’s a detailed overview:
„now“: Maps to DateTime.Now
, retrieving the current local date and time.
print(now())
„add_hours_to_date“: Maps to AddHours
, enabling you to add a specific number of hours to a date object.
future := add_hours_to_date(now(), 5);
print(future);
„try_parse_date“: Maps to DateTime.TryParse
, attempting to parse a string into a DateTime
object without throwing an exception.
date := try_parse_date("2025-02-04");
print(date);
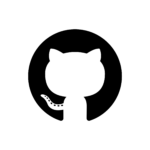
Looking for the
Github Repository?
The LUC compiler and tools are all written in LUC, with most development taking place on GitHub. Be sure to watch the repository to get updates on LUC’s development, or star it to show your support.