Calculator Application with LUC
The following code defines a simple calculator function, simple_calc
, which performs basic arithmetic operations based on a given operator. The function supports addition and subtraction, returning the result of the operation or 0
if an invalid operator is provided.
function int simple_calc(string sign, int first, int second){
if sign == "+" {
r first + second;
}
else if(sign == "-") {
int result = first - second;
r result;
}
else {
return 0;
}
}
function Main(){
resPlus := simple_calc("+", 10, 20);
resPlusString := to_string(resPlus);
print(resPlus);
resMinus := simple_calc("-", 5, 2);
string resMinus = to_string(resMinus);
print(resMinus);
print("Done");
}
The simple_calc
function takes three parameters: an operator (sign
) as a string and two integers (first
and second
). It checks the operator and performs the corresponding calculation. If the operator is "+"
, it returns the sum of the two numbers. If it is "-"
, it calculates the difference and returns the result. Any other operator results in returning 0
.
The Main
function demonstrates the usage of simple_calc
by performing an addition and a subtraction. The results are converted to strings and printed. Finally, the program prints "Done"
to indicate completion.
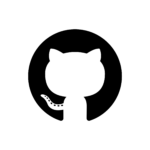
Looking for the
Github Repository?
The LUC compiler and tools are all written in LUC, with most development taking place on GitHub. Be sure to watch the repository to get updates on LUC’s development, or star it to show your support.