Overview of LUC Programming Constructs
Overview
This page provides an overview of key programming constructs in LUC, including loops, conditionals, and exception handling.
You’ll learn how to iterate through collections, control program flow with conditions and loops, and handle errors gracefully.
Whether you’re familiar with other languages or new to LUC, this guide will help you understand how to structure your code for efficiency and clarity.
Create Variable
In LUC, variables store values that can be used throughout the program. The following statement demonstrates how to declare an integer variable:
int first = 10;
This means that the variable first
in LUC now holds the number 10
, which can be used later in operations, functions, or other parts of the program.
Variable Decleration in LUC
In LUC, variables can be declared in two ways:
Way 1: Explicit Type Declaration
string test = "hello world";
Way 2: Type Inference (Shorter Syntax)
test := "hello world";
Both approaches achieve the same result—storing "hello world"
in the variable test
—but the second method offers a more concise syntax.
Function Declaration in LUC
In LUC, functions can be declared with or without a return type.
Explicit return type:
function int test() {
// Function body
}
Optional Return Type (Void Function)
function test() {
// Function body
}
- If no return type is specified, the function does not return a value.
- This is useful for functions that perform actions without needing to return data.
Both methods allow for flexible function creation in LUC.
Main Function in LUC
In LUC, the Main
function serves as the entry point of the program. It is where execution begins.
Decleration of the the Main Function
function Main() {
// Program execution starts here
}
Since no return type is specified, Main
does not return a value. If needed, additional functions can be called inside Main
to execute various tasks.
List Creation in LUC
In LUC, lists can store multiple values of a specific data type.
Example: Creating an Integer List
testList[int] = [10, 20, 40];
This creates a list named testList
containing the values 10, 20, and 40. Other data types (e.g., string
, float
) can be used similarly.
Dictionary Creation in LUC
In LUC, dictionaries store key-value pairs, where each key maps to a corresponding value.
Explicit Type Decleration
my_dictionary{string, int} = {test: 10};
Type Inference (Shorter Syntax)
my_dictionary := {test: 10};
Both methods create a dictionary where "test"
is mapped to the value 10
.
Looping
LUC provides several ways to control the flow of execution using loops, conditionals, and exception handling.
Foreach Loop
for(list) {
// Code to execute for each item
}
Example: Iterating Over a List of Words
for(string word in words) {
print(word);
}
- Iterates through each
word
in the listwords
. - Prints each word.
Simple For Loop
Used when the number of iterations is known.
for i < 20 {
print(i);
}
Iterates from an implicit starting point until i
is less than 20
.
Full For Loop (With Initialization, Condition, and Increment)
for int i = 0; i < 20; + {
print(i);
}
While Loop
Executes as long as the condition is true
.
while(test < 10) {
test += 1;
}
- Continues looping while
test
is less than10
. - Increments
test
each time.
If Condition
Executes a block of code if the condition is true
.
if(test == "test") {
print("Condition met!");
}
- Checks if
test
is equal to"test"
. - If
true
, prints"Condition met!"
.
Exception Handling
LUC handles errors with a simple syntax.
Single-Line Try-Catch
print("Hello world"); ? print("An error occurred");
Multi-Line Try-Catch
{
print("Hello world");
print("this");
} ? {
print("An error occurred");
print("That is very sad");
}
- The first block executes if no error occurs.
- If an error happens, the second block executes instead
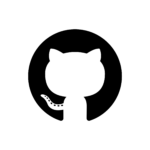
Looking for the
Github Repository?
The LUC compiler and tools are all written in LUC, with most development taking place on GitHub. Be sure to watch the repository to get updates on LUC’s development, or star it to show your support.